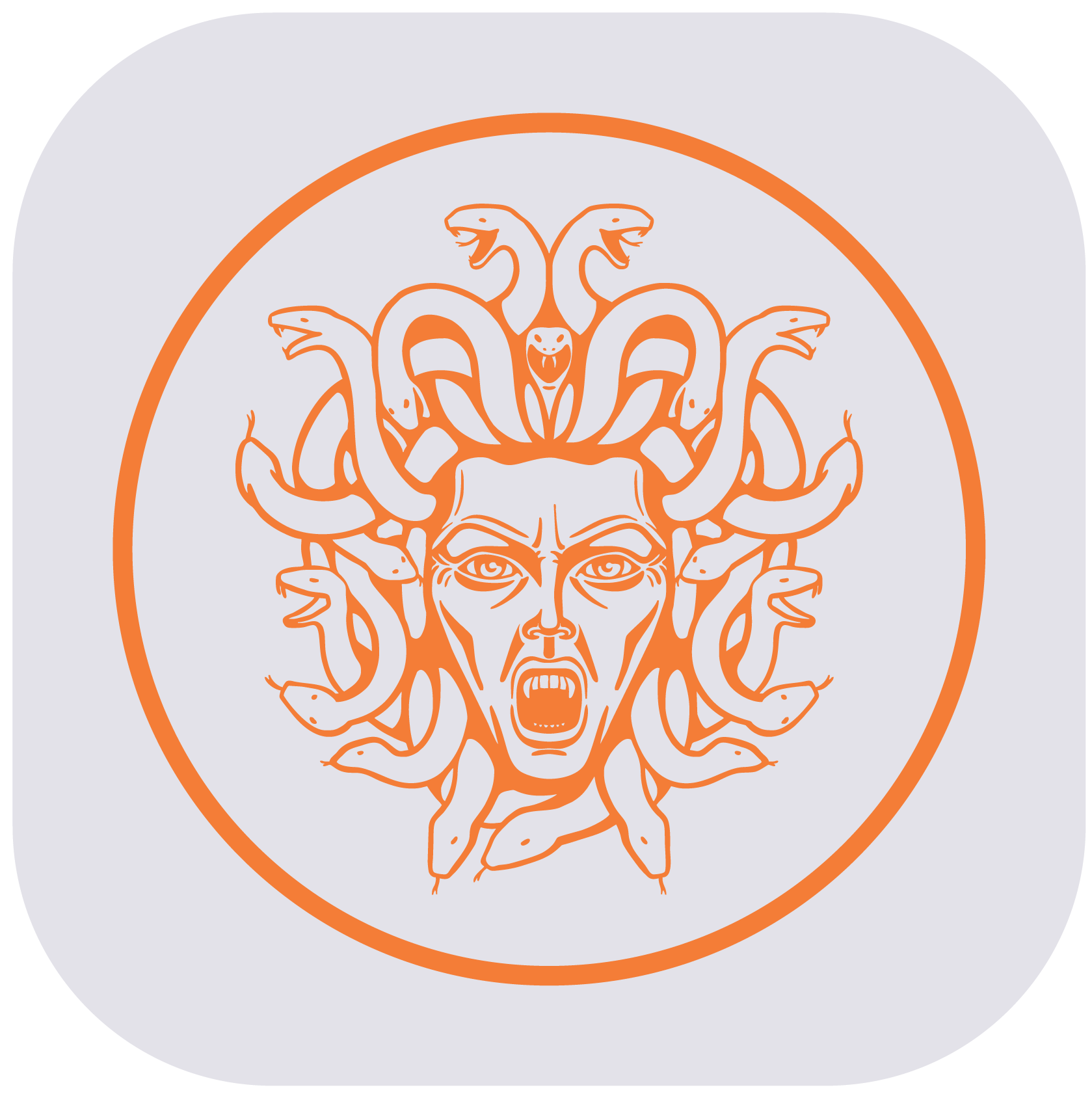
A javascript library to simplify caching data
3 min read
Gorgon.js
For a few years now I have maintained a project called medusajs. I haven’t needed to update it in a long time, and I finally think it’s time.
For this update I’m going to move the library to typescript as that is now building projects and it will be very easy to convert existing Medusa installs to use Gorgon.
I have created a proper modern OSS library website for this one: https://gorgonjs.dev please check it out
Gorgon also lets us send in full async functions instead of promise resolvers and a full quire of typescript types:
// file concerts.ts
import Gorgon from '@gorgonjs/gorgon';
const concertsGet = async (artist: string) => {
return Gorgon.get(`concerts/${artist}`, async ():Promise<Array<any>> => {
return fetch(`https://example.com/concerts/${artist}`)
.then(r => r.json());
}, 1000 * 60 * 60) // 1 hour
};
const concertsAdd = async (artist: string, concerts: any[]) => {
const concertDetails = await concertsGet(artist);
concertDetails.push(...concerts);
await fetch(`https://example.com/concerts/${artist}`, {
method: 'POST',
body: JSON.stringify(concertDetails),
});
Gorgon.clear(`concerts/${artist}`);
}
const concertsInvalidate = async (artist?: string) => {
if (artist) {
Gorgon.clear(`concerts/${artist}`);
} else {
Gorgon.clear(`concerts/*`);
}
};
export { concertsGet, concertsAdd, concertsInvalidate };
Medusa.js
So what does this mean doe medusa.js? for now the plan is to update the npm page and github to indicate that a newer better library exists, but I will continue to make any patches to the software. I still use this in some older projects and I would hate to have to force someone to switch libraries.
That said, any new additions are going to go into Gorgon
What’s next?
Modern Javascript / Typescript libraries tend to include plugin support for teh most popular apps and frameworks, so I’m going to be working on that. I hope to have a useGorgon
setup for React later this week and to at the very least add some sample projects to the repository for a bunch of other frameworks in the coming weeks.
Update: I actually finished up teh basic useGorgon hook for React, so you can check that out here: https://gorgonjs.dev/docs/ui/react